It is one of the most common issue newbie developers face while starting with java programming . What does this error mean could not find or load main class in java code.
Let’s take classic HelloWorld example . We have a HelloWorld.java class and I am trying to run the code from command line . This issue is seen mostly when java is not able to find the class it is trying to execute in your CLASSPATH .
In order to fix this error you need to have clear understanding of How Java class loading Works and working of CLASSPATH. For our understanding we won’t go deep into CLASSPATH and class loading .
So for Understanding CLASSPATH is basically a part of our environment variable , While running the Java file if the corresponding .class file is not found on CLASSPATH , java will throw this error : could not find or load class Main . Where Main is the name of the class.
So new Java developers uses lot of IDE like eclipse and intellij to run and debug Java code . It is easier to run and debug java code in IDE than command line . In eclipse or IntelliJ if you get this error you will not be able to understand the reason quickly if you are new java developer .
Have a Look at few Best Spring Courses for Java Developers in 2021
As IDE itself manages all the CLASSPATH
related stuff internally , but while you start running the same code from your terminal in linux or windows command line you will actually face this issue due to CLASSPATH
.
So now java CLASSLOADERS scans through the classpath to load your classess. Now lets come back to the problem where we get Could not find or load main class.
How do We fix the Error: Could not find or load main class HelloWorld
I bet you this troubleshooting that we are doing here to resolve the issue related to loading main class is going to help you in your future , till you are Java developer . So we will now Write a Simple HelloWorld program and replicate the issue , then we will try to fix it .
package frugalis;
public class HelloFrugalis
{
public static void main(String args[])
{
System.out.println("Welcome , Error Resolved!!");
}
}
Now move the above java file in a folder named as myProject
. We will now compile and try to run the code from command line .
mkdir myProject

Bingo !! We have got the error “Error: Could not find or load main class”.
Now , Why this is happening . We have knowingly defined the package as frugalis in our java code and we are trying to execute it from myProject
folder . Sounds some issue rite ? Lets try and fix it .
We are going to create a folder named as frugalis
inside MyProject
like below .
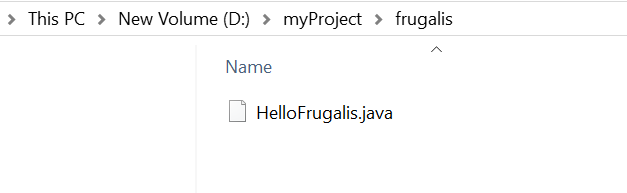
Now once you have added the file under folder frugalis
, it perhaps aligns with the package name and this should run successfully. Let’s try and run this .
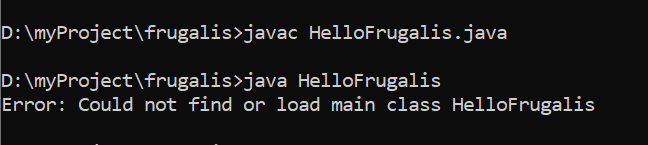
So again we are getting same Error: Could not find or load main class . So the problem here is my class is inside non-default package ex:- frugalis
in this case. We do not have any CLASSPATH variable due to which it is looking for path as frugalis/HelloFrugalis
from current directory .
But we are already inside the directory where .classfile
is present , hence we need to move to parent directory and execute the java class. My parent directory is myProject
.

Awesome !! It Works fine now !!!
So the reason it works fine now is in java World one we place a . it looks in current directory and resolves it like ./frugalis/HelloFrugalis
. In this is case it is able to locate our .class
and hence the code run successfully.
If you are a beginner and just started your Programming on Java , or someone who is not able to understand java .Follow the book Head First Java , One of the Best book.
Now lets tweak the problem a bit More Complex.
What if We want to Run the Above Code from a Separate Directory let’s say in the above example E:
drive instead of D:
drive . My Code remains in the same location , but I want to execute the code from a different location .
Well , in order to achieve this you need to set CLASSPATH by either -classpath
or -cp
option in CommandLine . It actually overrides the CLASSPATH environment variable as well .
Now lets run the code from a different directory
E:\>java frugalis.HelloFrugalis
Error: Could not find or load main class frugalis.HelloFrugalis
Again we are getting the same error , because java is looking in the working directory which is E:
in my case , there are no .class file with resolved path as E:\frugalis\HelloFrugalis . Now with CLASSPATH option , we will ask java to look at the class file from other directory .
Lets see how we can use CLASSPATH to solve the above issue .
java -cp D:\myProject; frugalis.HelloFrugalis
Welcome , Error Resolved!!
It runs successfully now , we have set classpath using -cp option for our java code and my classpath is pointing to D:/myProject
where my classfile is present .
Now , in case you have created the Project using any IDE like eclipse or IntelliJ , you will have a target folder where your packages and class files will be present . So target will act as a parent directory .
There are numerous reason due to which Error: Could not find or load main class HelloWorld error is shown . If you are working with lot of external Jars you can also get the error Could not find or load main class in jar as well. This is probably occurring as your jar is not placed in classpath .
But if you understand the basics of CLASSPATH and how it resolves the .class files in Java . You will be able to debug and dig further .
In 99% scenarios where you get this kind of error it is definitely a problem with your CLASSPATH environment variable in popular IDE or you are providing wrong location of your .jar or.class
files using -classpath
or -cp
options .
Fixing Error: Could not find or load main class in Spring boot
In this case , you will mostly get if you are running via any IDE . If you have any confusion make sure you try and run your spring boot project simply using
mvn spring-boot:run
Once the above command gets executed successfully . Now you navigate back to eclipse and try refreshing the project once .
This will load all the compiled classess in classpath and your application should work fine now .
Conclusion :-
As you are aware it can happen due to number of reasons , lets summarize in short and what we could do .
- If you are running your program from a directory where you have your code as well then make sure you are not executing your code from the location where .class file is present instead run it from parent directory .
- Set CLASSPATH environment variable and then try running your code from different locations . You need to set CLASSPATH for java lib as well if you are using java related library from console .
- If you are running a Java based application from Eclipse or Intellij and you are getting the Error:Could not find or load main class . Make sure your CLASSPATH Environment variable is correctly set or your JDK home is added properly in classpath . Most of the time it has been noticed that JRE home is set incorrectly , i would suggest setting JDK location.
- If you are running a Spring Boot based application and you are getting could not find or load main class in spring boot . Make sure your maven is building the classes properly and Java Home is set properly.
- Exception in thread “main” java.lang.NoClassDefFoundError: HelloWorld it also occurs more or less due to same reason . But it happens if you are using latest JDK over 1.6 .
I have tried to provide a quite a lot of suggestions and shown with one example to solve it , if still you guys are facing it let us know in the comments below …..
Happy Coding …………………
Also Read